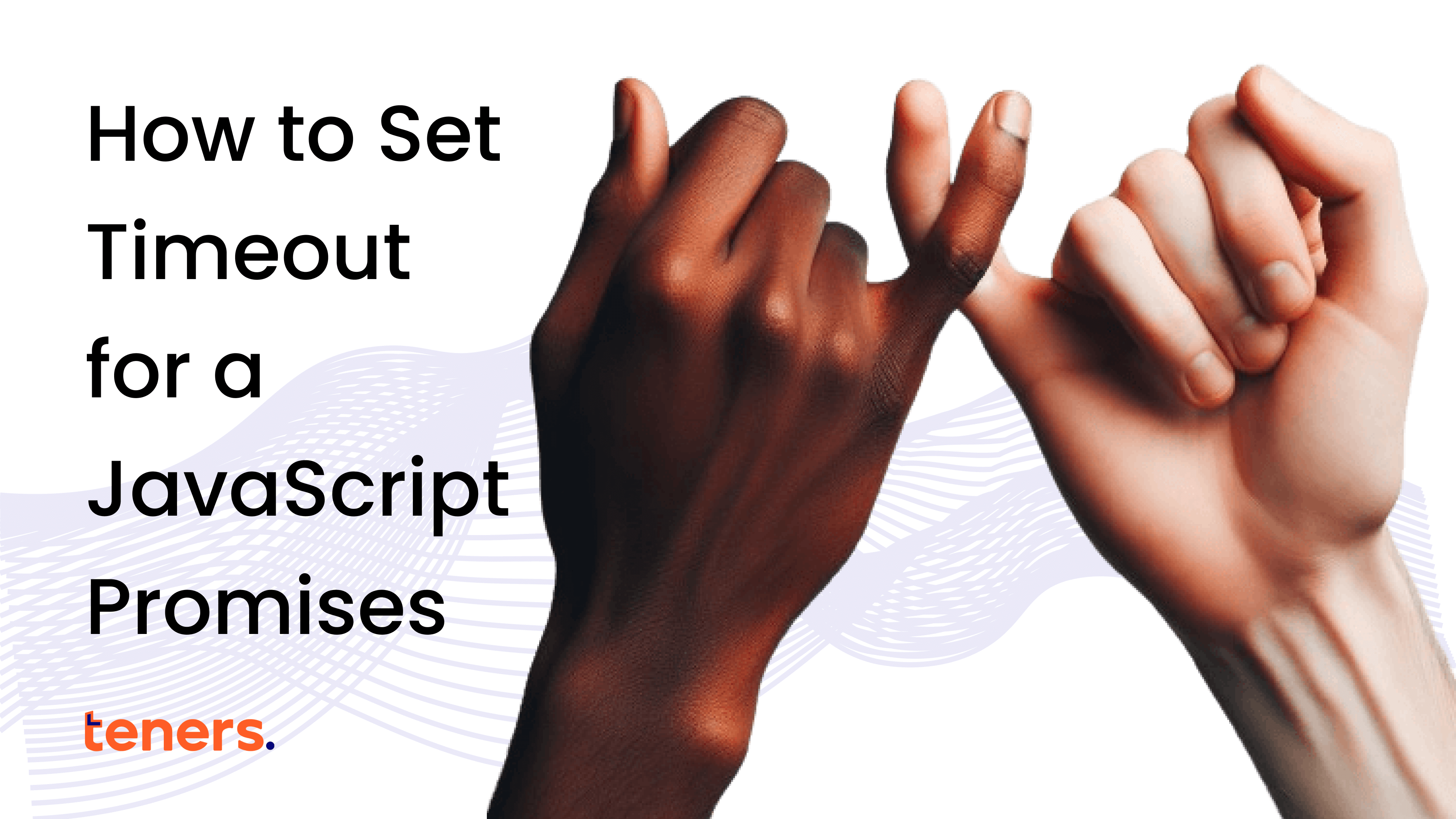
JavaScript promises are a powerful tool for handling asynchronous code execution. They allow us to write cleaner and more maintainable code by making it easier to handle async operations. However, there are times when we want to set a timeout for a promise to make sure it doesn't take too long to resolve or reject. In this article, we will explore different approaches to setting a timeout for a JavaScript promise and discuss the pros and cons of each.
Approach 1: Using Promise.race
One way to set a timeout for a promise is by using the Promise.race
method. This method takes an array of promises and returns a new promise that is settled as soon as any of the input promises are settled. We can leverage this behavior to create a timeout mechanism. Here's an example:
function timeoutPromise(promise, timeout) {
const timeoutPromise = new Promise(
(resolve, reject) => {
setTimeout(() => {
reject(new Error('Promise timed out'))
}, timeout)
}
);
return Promise.race([promise, timeoutPromise]);
}
// Usage
const promise = new Promise((resolve, reject) => {
// some async operation
});
const timeout = 5000; // 5 seconds
const result = await timeoutPromise(promise, timeout);
In this example, we create a new promise called timeoutPromise
that rejects after the specified timeout. We then use Promise.race
to return a new promise that settles either with the result of the original promise or with a timeout error, whichever occurs first.
Approach 2: Using async/await and setTimeout
Another approach is to leverage the power of async/await
syntax along with setTimeout
to create a timeout mechanism for a promise.
function timeoutPromise(promise, timeout) {
return new Promise(
async (resolve, reject) => {
const timeoutId = setTimeout(() => {
clearTimeout(timeoutId);
reject(new Error('Promise timed out'));
}, timeout);
try {
const result = await promise;
clearTimeout(timeoutId);
resolve(result);
}
catch (error) {
clearTimeout(timeoutId);
reject(error);
}
}
);
}
// Usage
const promise = new Promise((resolve, reject) => {
// some async operation
});
const timeout = 5000; // 5 seconds
const result = await timeoutPromise(promise, timeout);
Here, we create a new promise that wraps the original promise. We use async/await
to handle the asynchronous nature of the original promise. We start a timer using setTimeout
, and if the timer expires before the original promise resolves or rejects, we reject the new promise with a timeout error. Otherwise, we clear the timer and resolve or reject the new promise based on the outcome of the original promise.
Both of these approaches achieve the goal of setting a timeout for a JavaScript promise. However, they differ in how they handle the timeout mechanism. In approach 1 we use a Promise.race
to race the original promise with a timeout promise, while approach 2 uses setTimeout along with async/await to create a more integrated and readable solution.
It's important to note that setting a timeout for a promise is not a guarantee that the underlying async operation will be canceled. It only ensures that the promise itself will settle within the specified timeout.
If necessary, you should handle the cleanup of any resources associated with the async operation separately.
Conclusion
Setting a timeout for a JavaScript promise can be achieved using different approaches. The choice depends on the requirements and style preferences of the project. Regardless of the approach you choose, adding a timeout to promises can greatly enhance the robustness and reliability of your asynchronous code.